VR Grab Component
This is a component which allows hands grab it's attach parent component.(Attach parent must be a child of primitive component: static mesh, skeletal mesh etc.)
Class name:
Include:
Type:
Lines of code:
UVR_GrabComponent
#include "VR/VR_Components/VR_GrabComponent.h"
Interactable Component
742
General Information
Description
VR Grab Component is a scene component for grabbing/interacting(See VR Hand).
Settings
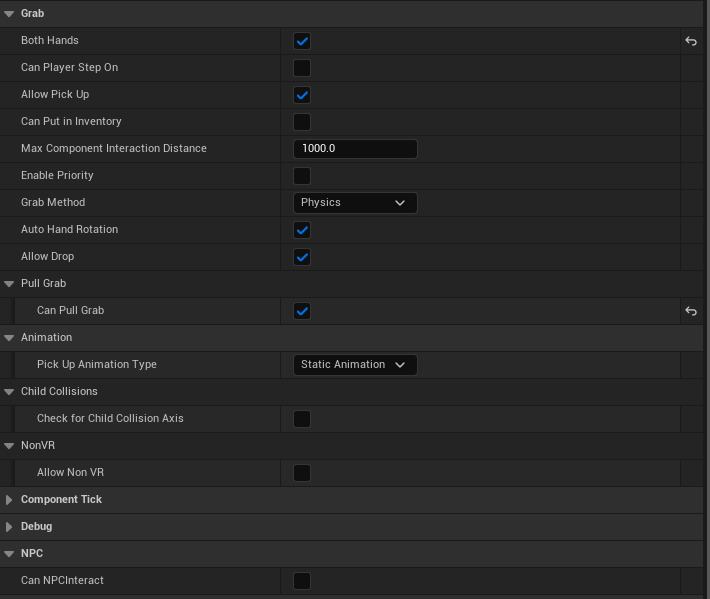
Can Put In Inventory - This variable indicates if player can put an actor to the hand inventory.
Grab Method - This variable indicates the grab method(See VR Variables). Each selection will bring additional parametrs such as animation variables, sockets etc.(In PhysicsAnimated and PhysicsAnimatedNearSocket, animation array index equals socket array index)
Allow Pick Up - This variable indicates if a player can grab the component.
Allow Drop - This variable indicates if a player can drop the component.
Both Hands - This variable indicates if player can the actor with both hands.
Can Player Step On - This variable indicates if player can step on this actor.
Can NPC Interact - This variable indicates if NPC can interact with this actor.
Max Component Interaction Distance - This is the max distance within which hand can grab.
Enable Priority - This prioritizes current grab component to be grabbed first among others.
Auto Hand Rotation - If set to true hand will be automatically rotated to fit the position.
Weld with hand - If true the component will be welded with a hand on grab.
Lower Attached Strength - If true physics constrraint force will be lower.
Can Pull Grab - This variable indicates if a player can pull grab this actor.
Grab Animation - This is hand grab animation variable which applyies on grab.
Trigger Animation - This is hand trigger press animation variable which applyies on trigger press.
A Pressed Animation - This is hand A press animation variable which applyies on A press.
B Pressed Animation - This is hand B press animation variable which applyies on B press.
Check For Child Collisions Axis - Checks if child collisions of UVR_GrabBoxCollisionComponent and UVR_GrabCapsuleCollisionComponent are attached to the component. If there are child collisions hand will be snapped to the nearest one.
Events
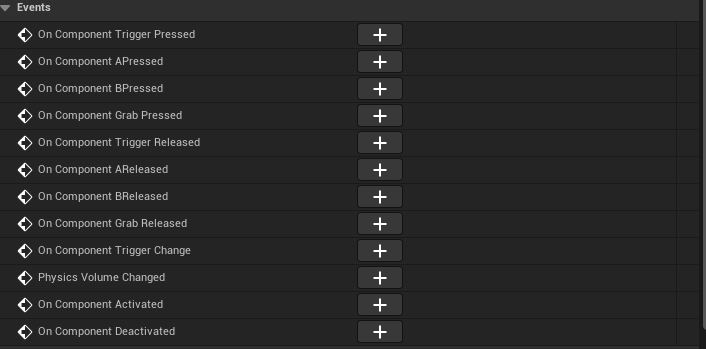
Blueprint API Reference
Public Member Functions | |
| |
| |
virtual bool | |
| |
virtual bool | |
| |
virtual bool | |
| |
void | OnHitCheckColliding (UPrimitiveComponent HitComponent, AActor OtherActor, UPrimitiveComponent *OtherComp, FVector normalImpulse, const FHitResult &Hit) |
| |
virtual void | TickComponent (float DeltaTime, ELevelTick TickType, FActorComponentTickFunction *ThisTickFunction) override |
| |
virtual void | Interaction_Implementation (class AVR_Hands *MotionController, bool &HandleStatus, bool bFroceAttachHandle) |
| Pickup Implementation. |
| |
virtual void | Stop_Interaction_Implementation (class AVR_Hands *MotionController) |
| Disconect object from Hand. |
| |
void | |
| Pickup object using AttachTo method Force attach , no physics. |
| |
void | |
| Pickup object using PhysicsConstraint method Physics , force attach. |
| |
void | |
| Pickup object using PhysicsConstraint method and animation.(Object snaps to taget) Physics , force attach. |
| |
void | |
| |
void | |
| Drops AttachTo method. |
| |
void | Drop_PhysicsConstraint (UPhysicsConstraintComponent *PickPhysicsConstraint) |
| Drops PhysicsConstraint method. |
| |
void | |
| Drops PhysicsConstraintAnimated method. |
| |
void | |
| |
void | ForceDrop () |
| Disconnects constraints. |
| |
virtual void | PickUpScript () |
| Executes before PickUp. Function can be overridden. |
| |
virtual void | DropScript () |
| Executes before Drop. Function can be overridden. |
| |
void | |
| Checks animation type for Drop_PhysicsConstraintAnimatedNearSocket. |
| |
void | SetGrabMethod (EGrabMethod NewGrabMethod) |
| Set Grab Method. |
| |
void | SetBothHands (bool BothHands) |
| If true player can pick an object using two hands. |
| |
void | EnablePullGrab (bool Enable) |
| |
void | EnableBlockPullGrab (bool Enable) |
| |
void | SetAllowPickUp (bool PickUpStatus) |
| Sets bSetAllowPickUp(If true player can Grab) |
| |
void | |
| Sets true for bJustDroped for a short time(Usefull if we need to put somthing in inventory). |
| |
FORCEINLINE EGrabMethod | GetGrabMethod () const |
| Get Grab Method. |
| |
FORCEINLINE UAnimSequence * | GetPickUpAnimation () const |
| Get PickUp Animations. |
| |
FORCEINLINE EAnimationType | GetAnimnType () const |
| |
FORCEINLINE bool | GetInHand () const |
| Get PickUp Animations. |
| |
FORCEINLINE bool | GetJustDroped () const |
| |
FORCEINLINE bool | CanPlayerStepOn () const |
| |
FORCEINLINE FName | GetLeftHandSocketName () const |
| |
FORCEINLINE FName | GetRightHandSocketName () const |
| |
FORCEINLINE uint8 | GetNumOfHandsAttached () const |
| |
FORCEINLINE bool | GetCanPutInInventory () const |
| |
FORCEINLINE bool | GetCanNPCInteract () const |
| |
FORCEINLINE bool | CanBothHandsGrab () const |
| |
FORCEINLINE int | GetGrabPriority () const |
| |
FORCEINLINE float | GetMaxInteractionDistance () const |
| |
FORCEINLINE bool | IsDropAllowed () const |
| |
FORCEINLINE bool | GetPullGrabEnabled () const |
| |
FORCEINLINE bool | IsAutoHandRotation () const |
| |
FORCEINLINE bool | IsCheckingChildCollisionAxis () const |
| |
FORCEINLINE bool | IsChildCollisionAxisInverted () const |
| |
FORCEINLINE EVectorDirectionTypes | GetChildCollisionDirectionAxis () const |
| |
bool | GetChildCollisions (TArray< UShapeComponent * > &ShapeComponents) |
| |
bool | InInteractionDistance (FVector Location, float &Distance) |
| |
bool | |
| |
void | |
| |
void | |
| On Collision Overlap Begin. |
| |
void | |
| |
void | APressed () |
| |
void | BPressed () |
| |
void | GrabPressed () |
| |
void | |
| |
void | AReleased () |
| |
void | BReleased () |
| |
void | GrabReleased () |
| |
void | TriggerChange (float Value) |
| |
void | |
| |
virtual void | BeginPlay () override |
| |
virtual void | EndPlay (const EEndPlayReason::Type EndPlayReason) override |
| |
| UPROPERTY (EditAnywhere, BlueprintReadWrite, Category="Grab|Animation", meta=(EditCondition="(GrabMethod == EGrabMethod::PhysicsAnimatedNearSocket || GrabMethod == EGrabMethod::AnimatedAndIK || GrabMethod == EGrabMethod::PhysicsAnimated) && PickUpAnimationType == EPickUpAnimations::Interactable", EditConditionHides)) UAnimSequence *PickAPressAnimation |
| |
| UPROPERTY (EditAnywhere, BlueprintReadWrite, Category="Grab|Animation", meta=(EditCondition="(GrabMethod == EGrabMethod::PhysicsAnimatedNearSocket || GrabMethod == EGrabMethod::AnimatedAndIK || GrabMethod == EGrabMethod::PhysicsAnimated) && PickUpAnimationType == EPickUpAnimations::Interactable", EditConditionHides)) UAnimSequence *PickBPressAnimation |
| |
| UPROPERTY (EditAnywhere, BlueprintReadWrite, Category="Grab|Animation", meta=(EditCondition="(GrabMethod == EGrabMethod::PhysicsAnimatedNearSocket || GrabMethod == EGrabMethod::AnimatedAndIK || GrabMethod == EGrabMethod::PhysicsAnimated) && PickUpAnimationType == EPickUpAnimations::Interactable", EditConditionHides)) UAnimSequence *PickTriggerPressAnimation |
| |
| UPROPERTY (EditAnywhere, BlueprintReadWrite, Category="Grab", meta=(EditCondition="(GrabMethod == EGrabMethod::Physics || GrabMethod == EGrabMethod::AnimatedAndIK)", EditConditionHides)) bool AutoHandRotation |
| |
| UPROPERTY (EditAnywhere, BlueprintReadWrite, Category="Grab|Child Collisions", meta=(EditCondition="(GrabMethod == EGrabMethod::Physics || GrabMethod == EGrabMethod::AnimatedAndIK)", EditConditionHides)) bool CheckForChildCollisionAxis |
| |
| UPROPERTY (EditAnywhere, BlueprintReadWrite, Category="Grab|Child Collisions", meta=(EditCondition="CheckForChildCollisionAxis == true && (GrabMethod == EGrabMethod::Physics || GrabMethod == EGrabMethod::AnimatedAndIK)", EditConditionHides)) bool InvertChildCollisionAxis |
| |
| UPROPERTY (EditAnywhere, BlueprintReadWrite, Category="Grab|Child Collisions", meta=(EditCondition="CheckForChildCollisionAxis == true && (GrabMethod == EGrabMethod::Physics || GrabMethod == EGrabMethod::AnimatedAndIK)", EditConditionHides)) EVectorDirectionTypes ChildCollisionDirectionAxis |
| |
Public Member Functions inherited from IVR_InteractionInterface | |
void | Pickup (class AActor VR_MotionConttroller, class USceneComponent MotionController, class UPhysicsConstraintComponent PickPhysicsConstraint, class USkeletalMeshComponent RealHandMesh, EControllerHand CurrentHand) |
| |
void | Drop (class UPhysicsConstraintComponent *PickPhysicsConstraint) |
| |
bool | IsGrabbed () |
| |
bool | CanPullGrab () |
| |
void | Interaction (class AVR_Hands *MotionController, bool &HandleStatus, bool bFroceAttachHandle) |
| Used for interaction with objects such as door. |
| |
bool | IsColliding () |
| |
void | Stop_Interaction (class AVR_Hands *MotionController) |
| Stop object interaction. |
| |
void | Shoot () |
| |
bool | |
| Indetify if object is held by MotionController. |
| |
bool | |
| |
bool | |
| |
void | NonVRInteraction (AVR_Player *Player) |
| |
bool | |
| |
void | Stop_NonVRInteraction (AVR_Player *Player) |
| Stop object interaction. |
|
Public Attributes | |
FComponentTriggerPressed | |
| |
FComponentAPressed | |
| |
FComponentBPressed | |
| |
FComponentGrabPressed | |
| |
FComponentTriggerReleased | |
| |
FComponentAReleased | |
| |
FComponentBReleased | |
| |
FComponentGrabReleased | |
| |
FComponentTriggerChange | |
| |
UPrimitiveComponent * | |
| |
bool | |
| |
bool | |
| |
bool | |
| |
bool | |
| |
bool | |
| |
float | |
| |
bool | |
| |
bool | |
| |
bool | |
| |
bool | |
| |
int | |
| |
| |
| |
UAnimSequence * | |
| |
bool | |
| |
FName | |
| |
FName | |
| |
TArray< FName > | |
| |
TArray< UAnimSequence * > | |
| |
float | |
| |
bool | |
| |
bool | |
| |
| |
| |
EControllerHand | |
| |
bool | bIsColliding = false |
| |
float | |
| |
class AActor * | |
| |
class USceneComponent * | |
| |
class UPhysicsHandleComponent * | |
| |
class UPhysicsConstraintComponent * | |
| |
class USkeletalMeshComponent * | |
| |
class USphereComponent * |